Jan
28
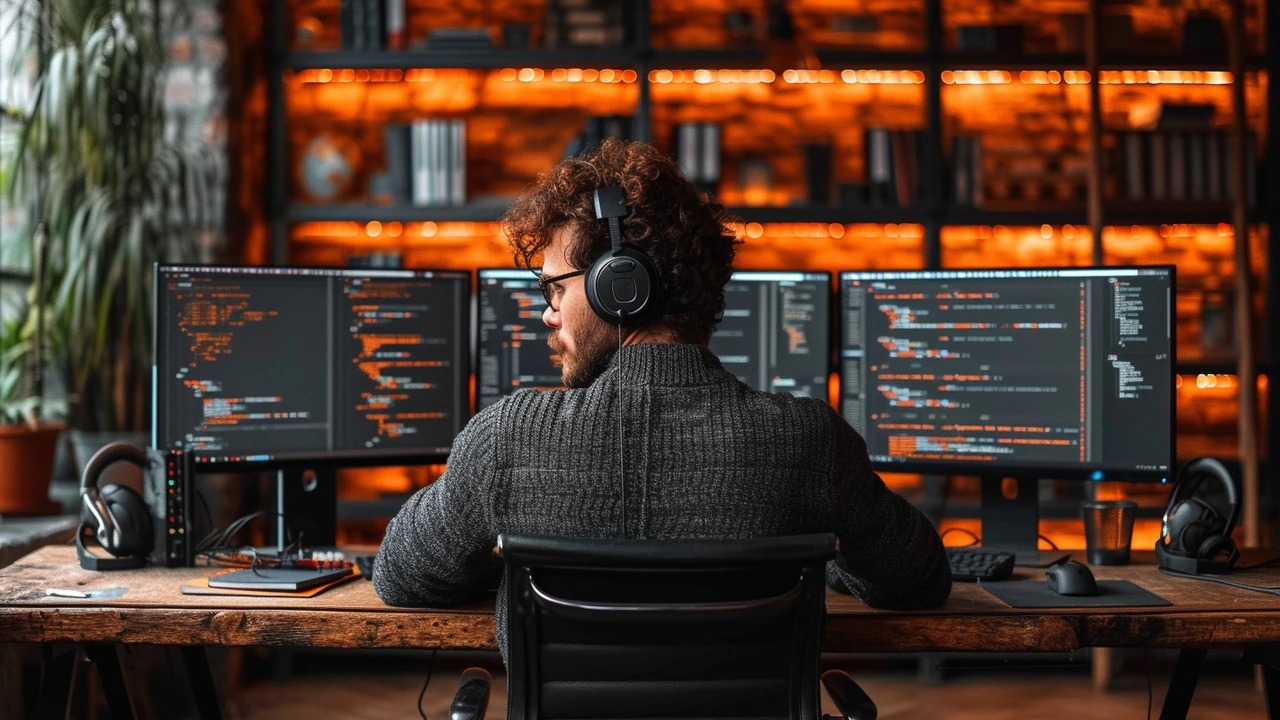
- by Miranda Fairchild
- 0 Comments
Efficient Use of Data Structures
When I first started with Python, the languages simplicity enraptured me. Its straightforward syntax and readability were like a breath of fresh air. However, as I delved deeper, I realized that mastery lies not just in understanding syntax but in effectively using Python's powerful data structures. Python offers a variety of data structures like lists, tuples, sets, and dictionaries, each with its own unique advantages. Understanding when and how to use these efficiently can drastically improve the performance of your code.
For instance, tuples, being immutable, can help in ensuring that data remains constant throughout the program. On the other hand, lists are mutable, making them ideal for storing collections of objects that need to be modified. Sets, with their unique ability to store non-duplicate items, are perfect for when you need to ensure the uniqueness of elements. Dictionaries, with their key-value pairs, offer unmatched speed for retrieval operations. Knowing when to leverage these structures can make all the difference.
Don't just stick to the basics; experiment with combining these data structures. Nested dictionaries, list comprehensions, and generator expressions are just the tip of the iceberg. Delving into these advanced uses opens up a plethora of possibilities, making your Python code not only more efficient but also cleaner and more readable.
Mastering Decorators and Generators
The power of Python also lies in its advanced features like decorators and generators. Initially, these concepts might seem daunting. However, with a bit of practice, they become indispensable tools in your Python arsenal. Decorators, for example, allow us to modify or enhance the behavior of functions or methods without permanently modifying their structure. This is particularly useful in implementing cross-cutting concerns like logging, authentication, or measuring execution time.
Generators, on the other hand, provide a way to iterate over data without the need to store it in memory all at once. This is especially beneficial when working with large datasets or streams of data. Mastering generators can lead to more memory-efficient and faster programs. Leveraging these two advanced features can significantly enhance the sophistication of your Python programs, allowing you to write more concise, efficient, and clean code.
Asynchronous Programming in Python
As applications grow more complex and I/O bound, the asynchronous features of Python become increasingly important. Asynchronous programming allows multiple operations to run concurrently, leading to more efficient use of resources and improved application performance. Python's async IO module is a game-changer in writing asynchronous code.
Understanding the event loop, coroutines, and futures can seem complex at first. However, learning these concepts allows you to handle I/O-bound and high-level structured network code more efficiently. Asynchronous programming in Python can take your skills to the next level, enabling you to build high-performance programs for web scraping, network communication, and more.
Optimizing Python Performance
Python is often criticized for its speed. However, with the right techniques, Python code can be optimized for performance. Profiling your code to understand where the bottlenecks lie is the first step. Tools like cProfile and timeit can help identify parts of the code that are slowing down your program.
Once bottlenecks are identified, techniques like using built-in functions, avoiding global variables, and minimizing the use of dots for attribute access can greatly enhance performance. For intensive computational tasks, libraries such as NumPy or even integrating with C can take performance to another level. Optimization is an art, and with practice, you can learn to write Python code that rivals the speed of more traditionally 'fast' languages.
Embracing Testing and Debugging
No code is perfect from the start. Testing and debugging are essential parts of the development process that ensure your code is reliable and robust. Python offers excellent tools like PyTest for testing and PDB for debugging. Writing tests may seem like extra work, but it saves time in the long run by catching bugs early.
Developing a habit of testing not only improves code quality but also helps in documenting how your code is supposed to work. Debugging, on the other hand, sharpens your problem-solving skills. Getting comfortable with these tools and practices makes you not just a better Python programmer but a better developer overall.
Staying Updated and Continuous Learning
The world of Python is ever-evolving. New libraries, tools, and best practices emerge regularly. Staying updated with the latest developments is crucial. Engaging with the community through forums, contributing to open source projects, or attending Python meetups and conferences can provide invaluable learning opportunities.
Remember, becoming an expert in Python programming is a journey. It requires patience, practice, and continuous learning. Take on projects that push your boundaries. Learn from your mistakes, and never stop experimenting. With time, the complexities of Python unravel, revealing a world of possibilities that can transform you into a Python maestro.
Write a comment