Jan
25
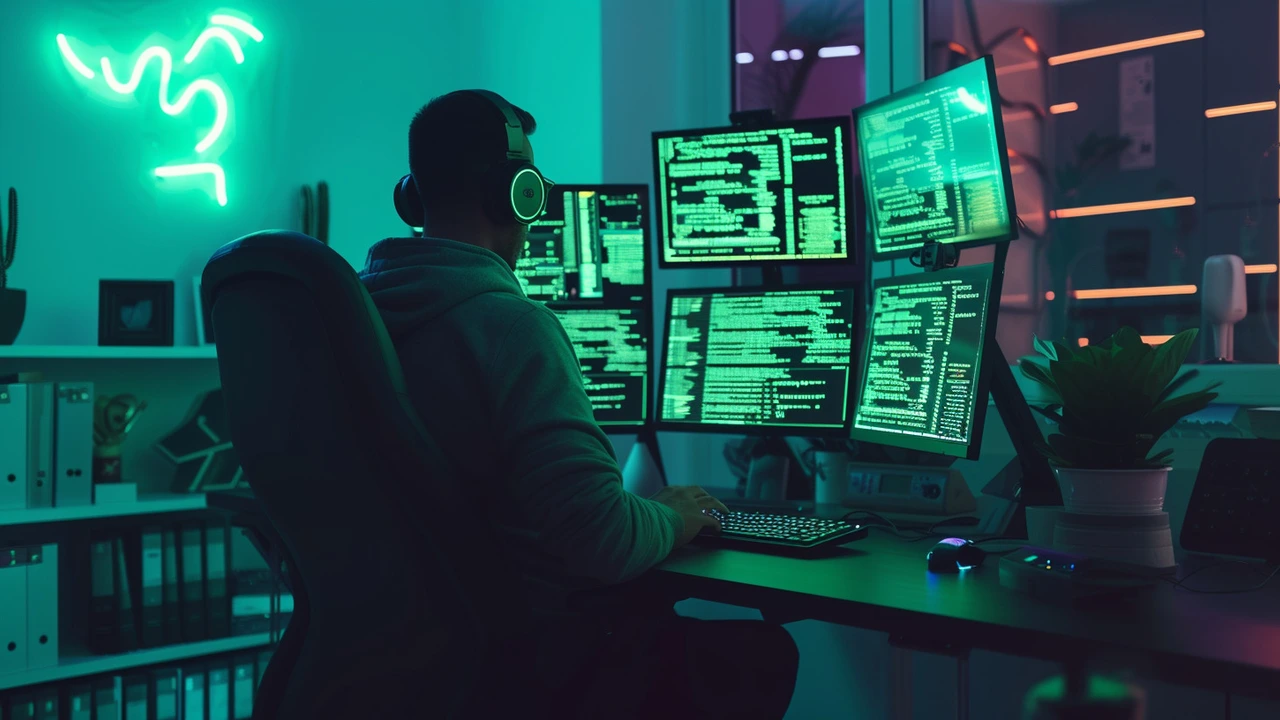
- by Elise Caldwell
- 0 Comments
Unlocking Python's Full Potential
When it comes to coding in Python, efficiency and simplicity often lead the way. It's a language beloved for its readability and ease of use, but don't let its straightforward nature fool you—there's immense power under the hood. Advanced programmers know that mastering the nuances of Python can lead to cleaner, faster, and more robust code. It's not just about what you code, but how you code it. Methods such as utilizing list comprehensions, embracing the power of the standard library, or harnessing decorators, can dramatically alter the performance and readability of your scripts. Let's delve into the wizardry of Python and equip ourselves with the knowledge to write code that even Guido van Rossum would be proud of.
The Zen of Python: Writing Readable Code
Pythonistas are familiar with the Zen of Python, which highlights the importance of code readability and simplicity. There's an art to writing code that not only computers but also humans can easily digest. Adopting naming conventions and adhering to PEP 8 standards isn't just for aesthetics; it enhances collaboration and long-term code maintenance. Strive for clarity over cleverness—your future self and fellow developers will thank you. Deliberate structuring and consistent style can save hours of debugging and can be the difference between a codebase that's a joy to work on and one that's a tangled web of complexity.
Automating the Boring Stuff
One of the greatest joys in scripting is automating mundane tasks. Whether you're renaming files in bulk, scraping a website, or crunching data sets, Python has the ability to turn what was once tedious into a task of trivial effort. Libraries like 'os' and 'shutil' for file operations, 'requests' and 'BeautifulSoup' for web exploration, and 'pandas' for data analysis, make these chores a breeze. Regular practice with these tools can lead to efficient habits that transform lengthy manual processes into single-line commands. It’s not just about saving time but also about reducing error rates and increasing reproducibility of results.
Mastering Python's Magic Methods
Magic methods are special methods that have double underscores at the start and end of their names. They're key to some of Python's most iconic features, like object creation, iteration, context management, and so much more. By overriding these methods, you can define how your classes should behave with standard operations such as 'len', 'repr', or arithmetic operators. Understanding magic methods can elevate your code from functional to phenomenal, allowing a deeper integration with Python’s syntax and an interface that feels native to the language itself.
Efficient Error Handling and Debugging
Even the best programmers write code that sometimes fails. When those times come, knowing how to effectively handle errors and debug is invaluable. Python’s error handling mechanism with 'try-except' blocks can safeguard your code from crashing, while also providing thoughtful feedback to users. For tracking down bugs, robust logging via the 'logging' module or the use of a debugger can make identifying the origin of problems less akin to finding a needle in a haystack. It’s through these careful practices that we craft resilient software that can stand the test of runtime adversities.
Optimizing Code for Performance
It’s not just about getting things done, it’s about doing them fast and efficiently. Python provides countless avenues for code optimization. Profilers like 'cProfile' can help you spot bottlenecks. Data structures from the 'collections' module can provide performance boosts. Understanding algorithmic complexity is key to writing efficient code that scales. Opt for generator expressions over list comprehensions for large datasets to save on memory usage. Python allows for micro-optimizations that, while might not be significant on their own, can cumulatively result in a significant performance uptick for your application.
Concurrent and Parallel Programming in Python
When faced with high-volume or computationally intense tasks, the sequential execution of scripts might not cut it. Thanks to Python’s 'threading' and 'multiprocessing' libraries, you can spread tasks across multiple threads or processes, tapping into the power of concurrency and parallelism. But tread lightly, as this power comes with complexity. Deadlocks and race conditions are just some of the pitfalls of concurrent programming. Yet, when done right, it can transform your scripts into high-performance machines, context-switching and distributing tasks to minimize wait times and fully utilize computational resources.
Write a comment