Dec
10
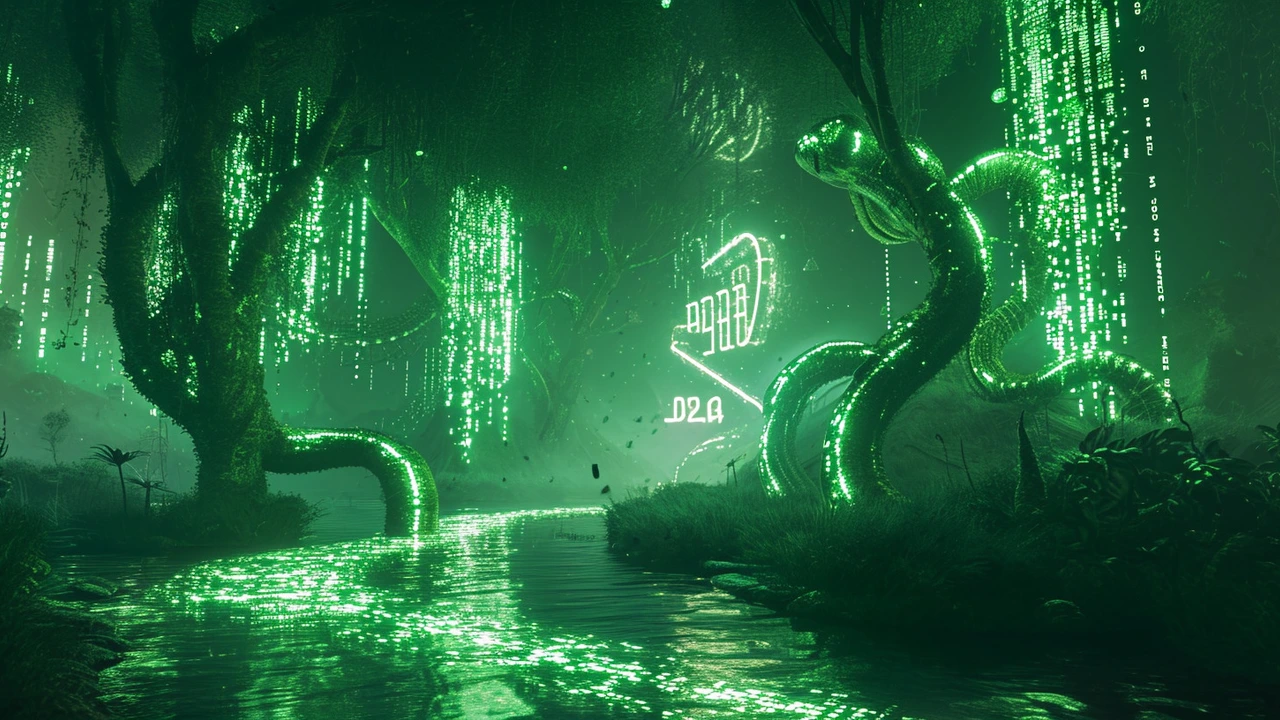
- by Warren Gibbons
- 0 Comments
Discover the Power of List Comprehensions
Here's a little secret to kick things off - Python list comprehensions. These are powerful constructs that can help save time, streamline code, and frankly, make you look like an absolute genius to your colleagues. Sounds good, right? Here's the lowdown.
Typically, you'd write a loop to generate a list, right? Well, with a list comprehension, you can do all this in a single line. It's the Python equivalent of a mic drop. It puts you on top of the programming world without breaking a sweat. Have you ever seen Emma, my wife, casually fit our entire family's weekly shopping into one car trip? It's that level of efficiency.
Picture this - you need a list of squares for the numbers 1 to 10. The old, traditional way would be a for loop. But with list comprehension, it's as simple as this: [i**2 for i in range(1,11)]. Efficiency personified.
Embrace the Magic of Lambda Functions
The term 'Lambda' might irk you, sounding like a complex equation straight from a nightmare maths quiz. I promise it's not. A lambda function is an anonymous, single-expression function that can be used anywhere function objects are needed. They’re the programming equivalent of one of those handy all-in-one tools you'd proudly display on your belt - I'm sure my son, Reid, will confirm.
Consider this situation - you need to write a function that takes two numbers and returns their sum. Normally, you’d write out a full function. But, with lambda, it’s as simple as lambda function: lambda x, y: x+y. With this, you've built a function in just one line. It's an impressive level of coding efficiency that's bound to turn heads.
Pick Up Regular Expressions for Data Mining
'Regular expressions’ or regex, a term that could easily get you mistaken for a coal miner. However, they are practical tools for pattern matching and data extraction and can be an awesome pickaxe in your data mining efforts. With regex, you can pull apart text, extract specific details, and even replace parts of the string. Don't believe me? Let's walk through it.
Consider this example – You have a text full of email addresses and you need to extract them. A regular expression can define the pattern of an email address, go through the text, identify all the matches, and poof! Extracted emails, simple as that. It's like my daughter Amity's knack for extracting every little detail about a pop star from a magazine feature.
Master the Zen of Python
The Zen of Python is a collection of 20 guiding principles for writing computer programs that influence the design of the Python Programming Language. While these aren’t technical, in-depth coding tips, they’re going to shape your approach to writing Python code moving forward.
The first principle, "Beautiful is better than ugly", implies that code should be aesthetic in addition to being functional. Make it clean, make it readable, make it pleasing to the eye. Remember the joy you felt when you first saw a well-organised bookshelf? It's that, but for code. Each principle brings a new perspective, fresh insights, and a whopping handful of wisdom to your Python programming.
Get Ahead with Decorators
Decorators are a powerful feature of Python that allows us to modify the behavior of a function or class without permanently changing its source code, much like if I could convince Emma to enjoy camping without the need for a five-star suite. It's a nifty way of adding to the functionality of your code without actually altering the core.
Imagine needing to add a logging feature or timing feature to multiple functions in your code. You could go and add the same lines in every function or you can simply create a decorator with these features and add it to the function. It’s uncomplicated, it's clean, and it's a great way to impress your colleagues with your advanced Python knowledge.
Expand with Modules and Packages
Python comes with a rich array of modules and packages which are sets of pre-written code you can use in your programs. Knowing about these modules is like having an entire toolkit at your disposal - you may not use every single tool every day, but the one day you need it, you'll thank yourself for having it ready.
There are modules for practically everything - networking, web scraping, date and time manipulation, and more. I swear, if there was a module for, say, folding laundry, Emma would download it in a heartbeat! Knowledge about these Python modules and packages will do wonders for your Python programming skills and seriously revolutionise the way you write code.
Tackle Python with the Assert Keyword
The assert keyword is a debugging tool that helps us to test conditions in our code. Think of it as your very own Python lie detector. If the condition is true, the program continues to run, but if it's false - the program stops, shines a light on the issue, and allows you to look into it.
It’s a great tool for catching bugs and making sure that your programs are running as expected. It’s like when my daughter, Amity, insists she’s done her homework. A quick check of her books - assert 'homework is done' - and we know the truth.
It might sound overwhelming at first, but with practice, your comprehension and application of these Python tricks will certainly elevate you to a whole new programming level. So, buckle up, and embrace the Python magic and soon you'll be impressing everyone with your code. Happy coding!
Write a comment